Chain of Responsibility パターン | デザインパターン
2022年9年25日
デザインパターン
Chain of Responsibility
PHP
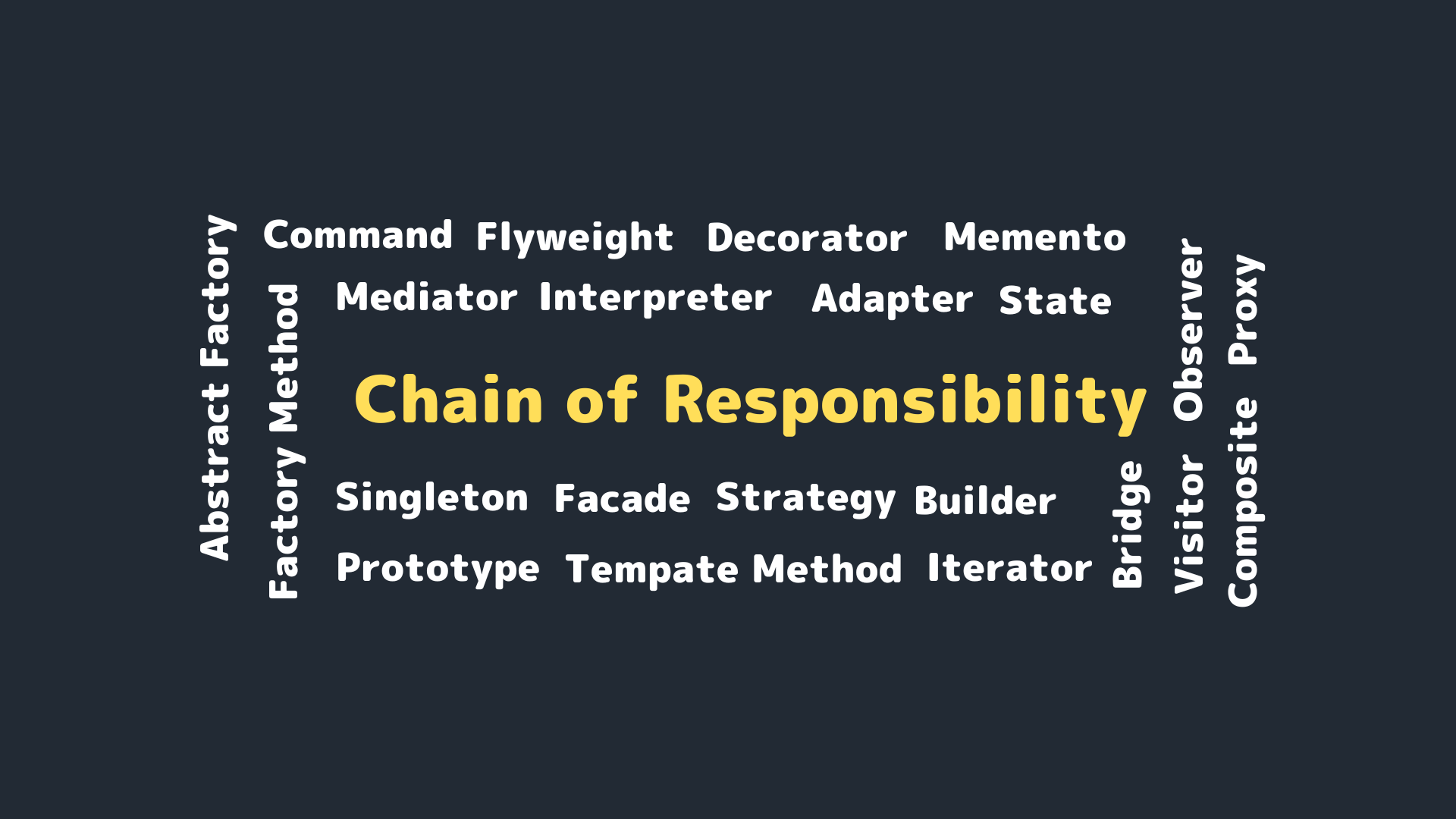
オライリージャパンによる Head First シリーズ デザインパターン(第2版)第14章 Chain of Responsibility パターンを、 PHPで書き直してみようという試みです。
Handler.php
namespace ChainOfResponsibility; abstract class Handler { public ?Handler $successor = null; public function setHandler(Handler $successor) : Handler { $this->successor = $successor; return $this; } abstract public function handleRequest(int $type) : void; }
SpamHandler.php
namespace ChainOfResponsibility; class SpamHandler extends Handler { public function handleRequest(int $type) : void { if($type == 0) { echo 'スパムメールに振り分けられました' . "\n"; } else if (!is_null($this->successor)) { $this->successor->handleRequest($type); } else { echo '検知されませんでした' . "\n"; } } }
FunHandler.php
namespace ChainOfResponsibility; class FunHandler extends Handler { public function handleRequest(int $type) : void { if($type == 1) { echo 'ファンメールに振り分けられました' . "\n"; } else if (!is_null($this->successor)) { $this->successor->handleRequest($type); } else { echo '検知されませんでした' . "\n"; } } }
ComplaintHandler.php
namespace ChainOfResponsibility; class ComplaintHandler extends Handler { public function handleRequest(int $type) : void { if($type == 2) { echo '苦情メールに振り分けられました' . "\n"; } else if (!is_null($this->successor)) { $this->successor->handleRequest($type); } else { echo '検知されませんでした' . "\n"; } } }
NewLocHandler.php
namespace ChainOfResponsibility; class NewLocHandler extends Handler { public function handleRequest(int $type) : void { if($type == 3) { echo '要望メールに振り分けられました' . "\n"; } else if (!is_null($this->successor)) { $this->successor->handleRequest($type); } else { echo '検知されませんでした' . "\n"; } } }
index.php
use ChainOfResponsibility\SpamHandler; use ChainOfResponsibility\FunHandler; use ChainOfResponsibility\ComplaintHandler; use ChainOfResponsibility\NewLocHandler; $spam = new SpamHandler(); $fun = new FunHandler(); $complaint = new ComplaintHandler(); $newLoc = new NewLocHandler(); $spam->setHandler($fun->setHandler($complaint->setHandler($newLoc))); $spam->handleRequest(0); // 0:スパムメール $spam->handleRequest(1); // 1:ファンメール $spam->handleRequest(2); // 2:苦情メール $spam->handleRequest(3); // 3:要望メール $spam->handleRequest(4);
出力結果
スパムメールに振り分けられました ファンメールに振り分けられました 苦情メールに振り分けられました 要望メールに振り分けられました 検知されませんでした