Decorator パターン | デザインパターン
2022年9年23日
デザインパターン
Decorator
PHP
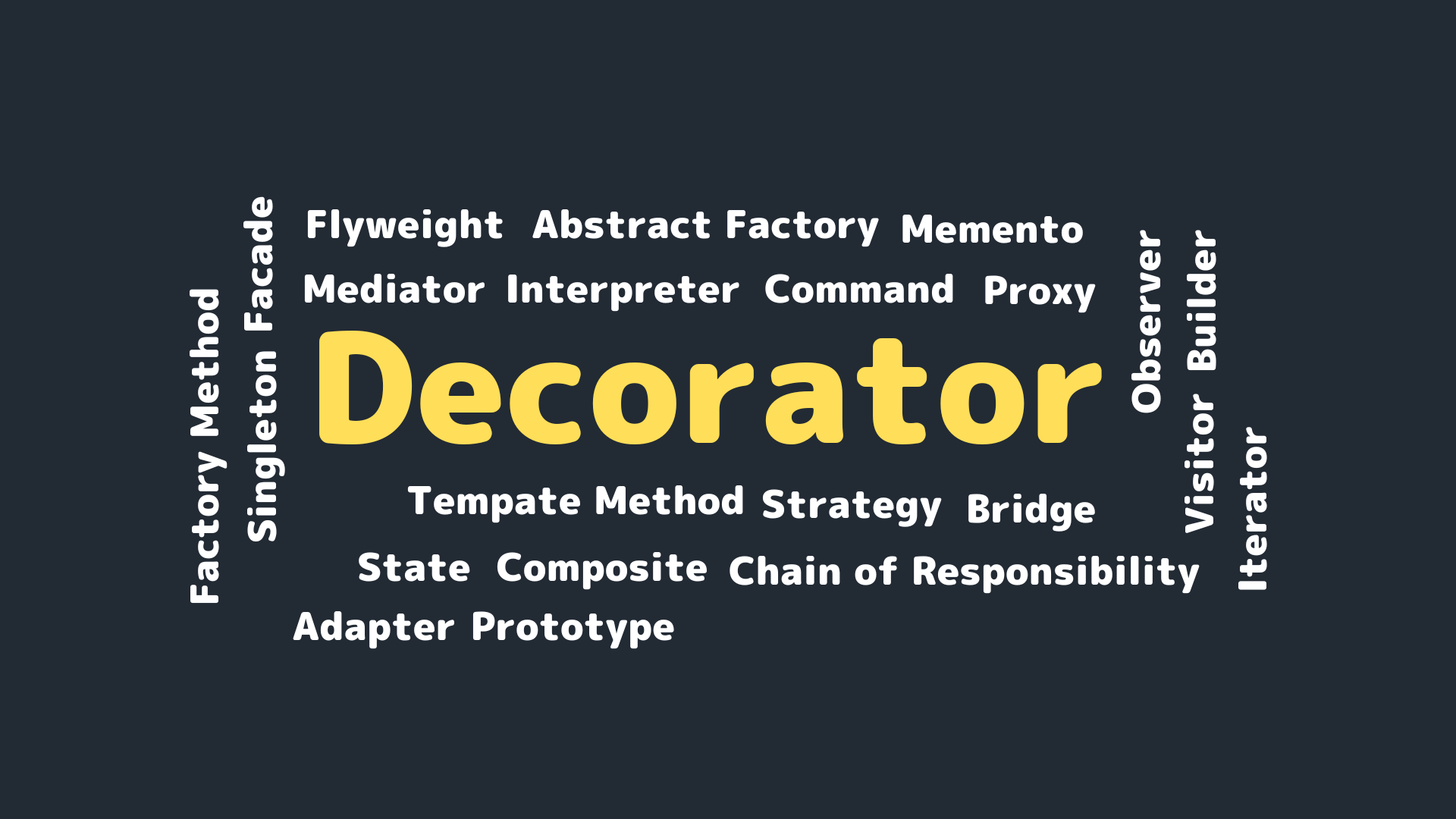
オライリージャパンによる Head First シリーズ デザインパターン(第2版)第3章 Observer パターンを、PHPで書き直してみようという試みです。
Beverage.php
namespace Decorator; abstract class Beverage { public string $description = '不明な飲み物'; public function getDescription() : string { return $this->description; } public abstract function cost() : float; }
Espresso.php
namespace Decorator; class Espresso extends Beverage { public function __construct() { $this->description = 'エスプレッソ'; } public function cost() : float { return 1.99; } }
DarkRoast.php
namespace Decorator; class DarkRoast extends Beverage { public function __construct() { $this->description = 'ダークローストコーヒー'; } public function cost() : float { return 0.99; } }
HouseCoffee.php
namespace Decorator; class HouseCoffee extends Beverage { public function __construct(){ $this->description = 'ハウスブレンドコーヒー'; } public function cost() : float { return 0.89; } }
CondimentDecorator.php
namespace Decorator; abstract class CondimentDecorator extends Beverage { public Beverage $beverage; }
Soy.php
namespace Decorator; class Soy extends CondimentDecorator { public function __construct(Beverage $beverage){ $this->beverage = $beverage; } public function getDescription() : string { return $this->beverage->getDescription() . '、豆乳'; } public function cost() : float { return $this->beverage->cost() + 0.15; } }
Mocha.php
namespace Decorator; class Mocha extends CondimentDecorator { public function __construct(Beverage $beverage){ $this->beverage = $beverage; } public function getDescription() : string { return $this->beverage->getDescription() . '、モカ'; } public function cost() : float { return $this->beverage->cost() + 0.20; } }
Whip.php
namespace Decorator; class Whip extends CondimentDecorator { public function __construct(Beverage $beverage){ $this->beverage = $beverage; } public function getDescription() : string { return $this->beverage->getDescription() . '、ホイップ'; } public function cost() : float { return $this->beverage->cost() + 0.10; } }
index.php
use Decorator\Espresso; use Decorator\DarkRoast; use Decorator\HouseCoffee; use Decorator\Soy; use Decorator\Mocha; use Decorator\Whip; $beverage = new Espresso(); echo $beverage->getDescription() . ' $' . $beverage->cost(); echo "\n"; $beverage2 = new DarkRoast(); $beverage2 = new Mocha($beverage2); $beverage2 = new Mocha($beverage2); $beverage2 = new Whip($beverage2); echo $beverage2->getDescription() . ' $' . $beverage2->cost(); echo "\n"; $beverage3 = new HouseCoffee(); $beverage3 = new Soy($beverage3); $beverage3 = new Mocha($beverage3); $beverage3 = new Whip($beverage3); echo $beverage3->getDescription() . ' $' . $beverage3->cost(); echo "\n";
出力結果
エスプレッソ $1.99 ダークローストコーヒー、モカ、モカ、ホイップ $1.49 ハウスブレンドコーヒー、豆乳、モカ、ホイップ $1.34