Iterator パターン | Java言語で学ぶ デザインパターン入門
2022年10年2日
Java言語で学ぶ デザインパターン入門
Iterator
PHP
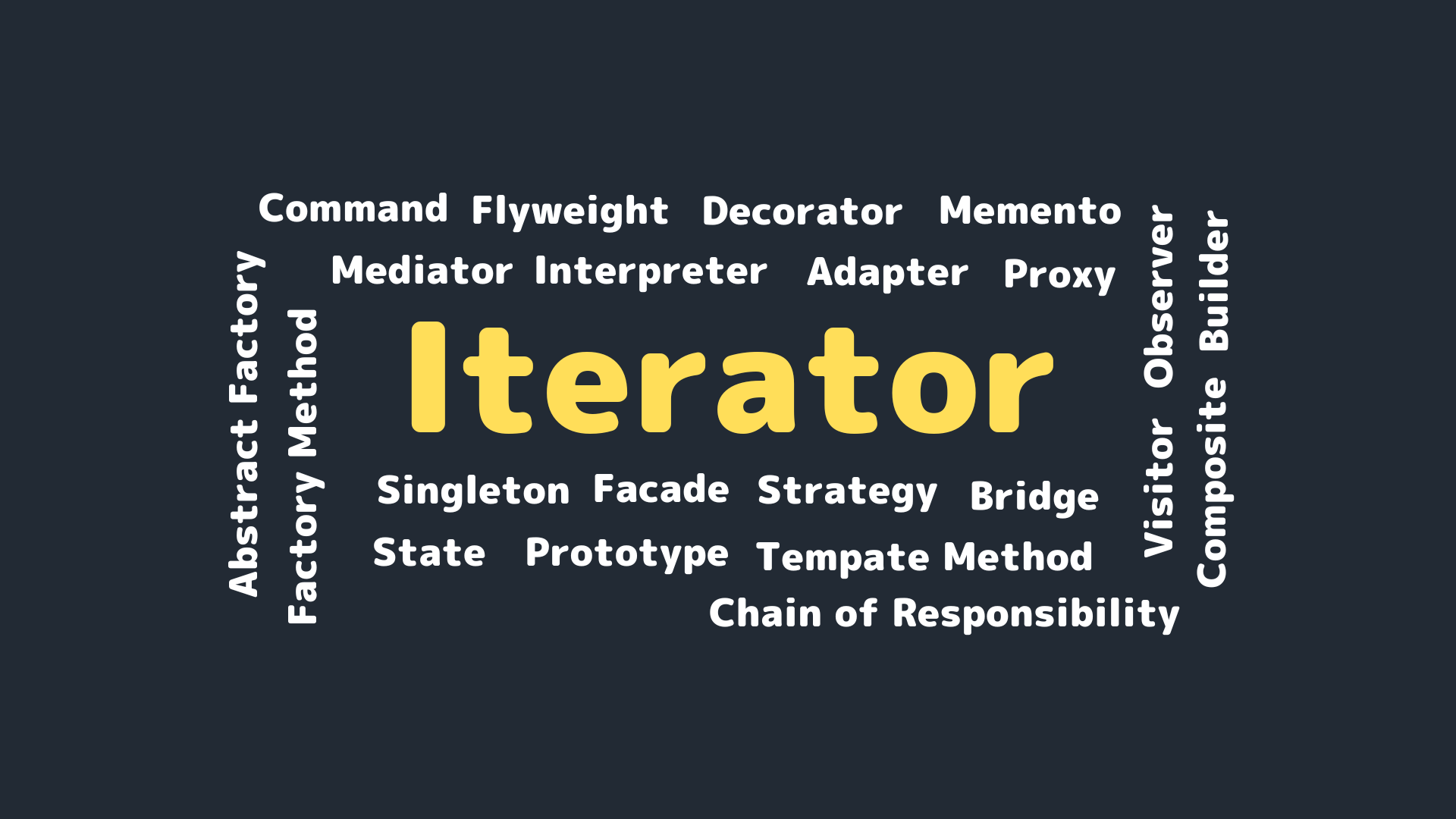
Java言語で学ぶデザインパターン入門(第3版)第1章 Iterator パターンを、PHPで書き直してみようという試みです。
Book.php
namespace Iterator; class Book { private string $name; public function __construct(string $name) { $this->name = $name; } public function getName() { return $this->name; } }
Aggregate.php
namespace Iterator; interface Aggregate { public function iterator(); }
BookShelf.php
namespace Iterator; class BookShelf implements Aggregate { public array $books = []; public int $last = 0; public function getBookAt(int $index) : Book { return $this->books[$index]; } public function appendBook(Book $book) : void { $this->books[$this->last] = $book; $this->last++; } public function getLength() : int { return $this->last; } public function iterator() { return new BookShelfIterator($this); } }
Iterator.php
namespace Iterator; interface Iterator { public function hasNext() : bool; public function next() : Book; }
BookShelfIterator.php
namespace Iterator; class BookShelfIterator implements Iterator { public BookShelf $bookShelf; public int $index; public function __construct(BookShelf $bookShelf) { $this->bookShelf = $bookShelf; $this->index = 0; } public function hasNext() : bool { if ($this->index < $this->bookShelf->getLength()) { return true; } else { return false; } } public function next () : Book { $book = $this->bookShelf->getBookAt($this->index++); return $book; } }
index.php
use Iterator\Aggregate; use Iterator\BookShelf; use Iterator\Book; use Iterator\Iterator; use Iterator\BookShelfIterator; $bookShelf = new BookShelf(); $bookShelf->appendBook(new Book('Around the World in 80 Days')); $bookShelf->appendBook(new Book('Bible')); $bookShelf->appendBook(new Book('Cinderella')); $bookShelf->appendBook(new Book('Dassy-Long-Legs')); $it = new BookShelfIterator($bookShelf); while ($it->hasNext()) { $book = $it->next(); echo $book->getName() . "\n"; }
出力結果
Around the World in 80 Days Bible Cinderella Dassy-Long-Legs